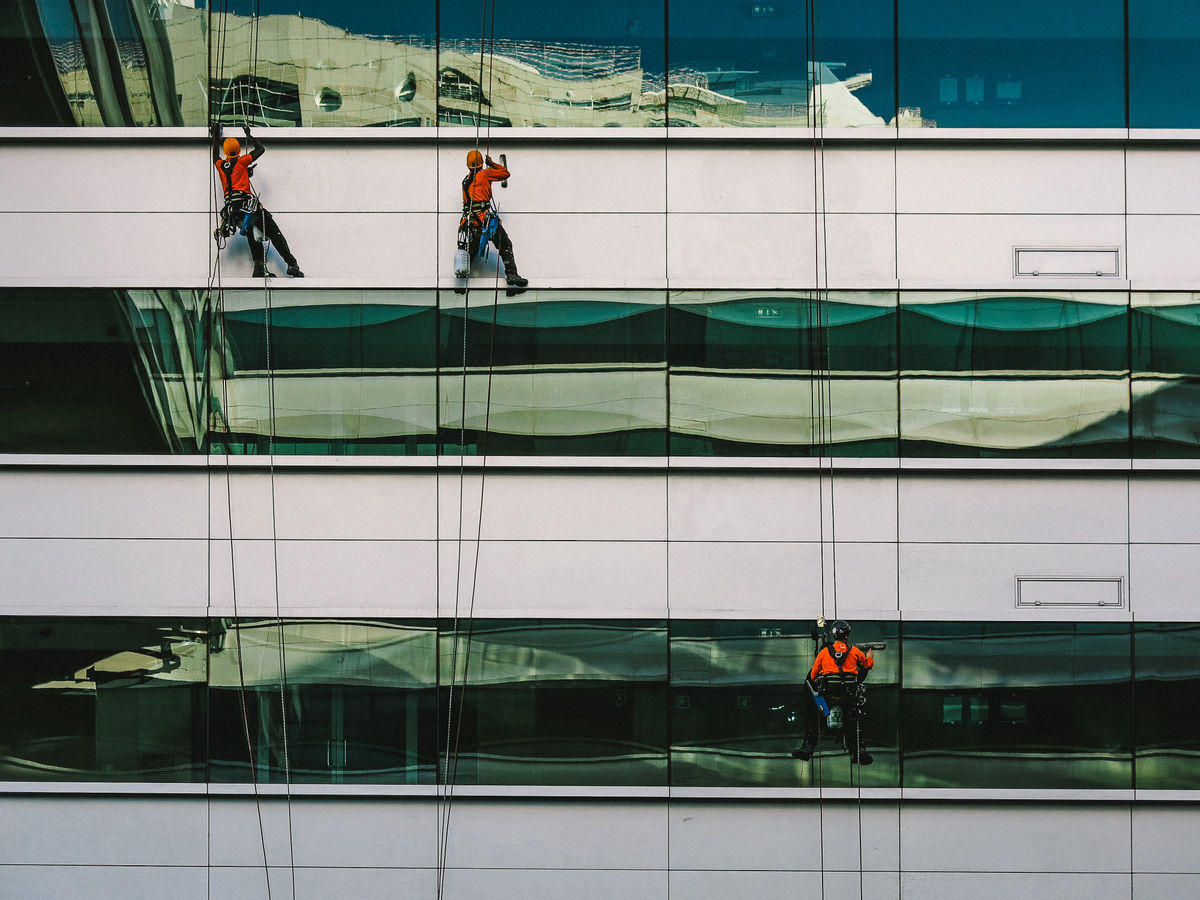
The pressure to build software quickly is great. Management wants features added yesterday. The competition is breathing down your neck and you must produce products to remain a relevant in your space. You cut a corner here, delay writing those automated tests over there. “It’ll just be faster if I bolt the feature on here,” you tell yousrelf. You promise to come back and do the necessary refactoring later.
Later never comes.
It’s like eating cake and cookies at every meal. They taste good at the time, but eventually your waistline expands and your health suffers. Eventually the technical debt catches up with you. It takes longer to implement seemingly simple things. The stability of the system begins to suffer and you spend more time keeping it running than adding new features. You may even reach the point where large portions of the system have to be scrapped and rewritten, at significant time and expense.
Software quality is not a bolt-on feature.
Code quality is something that must be considered at the earliest stages of a software project. Building it right the first time means that you can spend more time adding features and less time fixing problems.
If you don’t have time to do it right, when will you have time to do it over? - John Wooden
But software development is an iterative process, right? We don’t always know everything the customer will want up front! Isn’t refactoring code rework?
Reworking code in response to new product requirements or a better understanding of the problem is much different than going back to fix problems that were known to exist when the software was written initially.
Develop a mindset of quality and you’ll serve your customers better and be more productive.
So what are the attributes of quality software?
Clean
Quality code is clean. This means that the software has been written using best practices with respect to design and language. Clean code is code that is easy to understand and easy to change. What does this mean in practice? Here are a few characteristics of clean code:
- Simple. Even complex systems can be broken down into small, simple, easy to understand components. This could be small, well named methods within a larger object. It could mean small, comprehensible objects within a larger system.
- Clear. The system uses descriptive names for methods and variables. It is formatted in a way to improve readability. The code almost reads like natural language, making it easier to understand.
- Decoupled. The easiest code to understand, modify and test is one that has as few dependencies as possible. Components need to interact with each other, but it should be an orchestration between lots of easy to understand components, rather than one, large, complex, hard to understand component.
All of these are fairly qualitative measures of code quality, this is where the creative, artistic side of coding comes in. But trying to explain to your manager or product owner that the code doesn’t feel clean enough isn’t a terribly compelling argument.
Fortunately there are quantitative measures you can use to determine the cleanliness of your code:
- Cyclomatic complexity. Measures the number of independent paths through your code to determine how complex the code is.
- Halstead cvomplexity measures. These forumulas measure several properties of your code in order to determine the effort, time, and estimated number of bugs within a program.
- Lines of code. The more lines of code within a particular class or method, the more complex it’s likely to be. Of course, simply cramming a bunch of code on to a single line defeats the purpose here, so use this one carefully.
Tested
It’s impossible to know if your code is working if you don’t test it. You can do this manually, but do you really want to do that every build? Computers are much better at performing repetitive tasks than we are, so we write automated tests.
Quality code has a suite of automated tests that are run every time the software is built and deployed. Having such a collection of tests provides several benefits:
- Verification. Obviously, automated tests make sure that the code does what you think it should. But just as important, it tests what the code should not do. That is, it includes negative tests which verify that invalid inputs are handled appropriately.
- Safety. In order to refactor or otherwise update the code, you need to know that the changes haven’t affected the public apis, breaking the expectations of callers of your code.
- Design. The act of writing tests will often expose design flaws. Difficulty writing a test may mean that the code should be reworked to make it easier to inject the dependencies or otherwise simplify the test.
Whatever test framework or style of tests you write, treat them with the same care as the “main” code. Your tests will exist as long as your code does. As your code evolves, so will your tests. Make them as easy to understand and maintain as the code it tests.
“If you let the tests rot, then your code will rot too. Keep your tests clean.” ― Robert C. Martin
Maintained
Very little code is written once, and never updated again. Technologies improve, business requirements change, or we learn more about the business domain. If you don’t continually revisit your code, it falls by the wayside. It becomes that code that nobody understands and nobody wants to touch for fear that it might break something critical.
Continue to refactor and improve your code. Keep learning about the business domain, improve your understanding of the technologies involved. Apply this knowledge to improve your code.
This isn’t to say you should change code for change’s sake, but whenever you are working on a particular part of the system, always leave things better than you found them.
As always, if you have automated tests, the risk of refactoring and changing code is much lower.
Conclusion
Creating quality code takes extra work an attention. But if you’re willing to take the time to keep your code clean, tested and maintain it over time, you can reap the benefits over time. I think you’ll find that your productivity actually goes up, your customers have a better experience and you and your team will be able to sleep better at night, knowing that your software it working as intended.
Discussion Question****: What do you think are the main software quality attributes?